Are strongly typed functions as parameters possible in TypeScript?
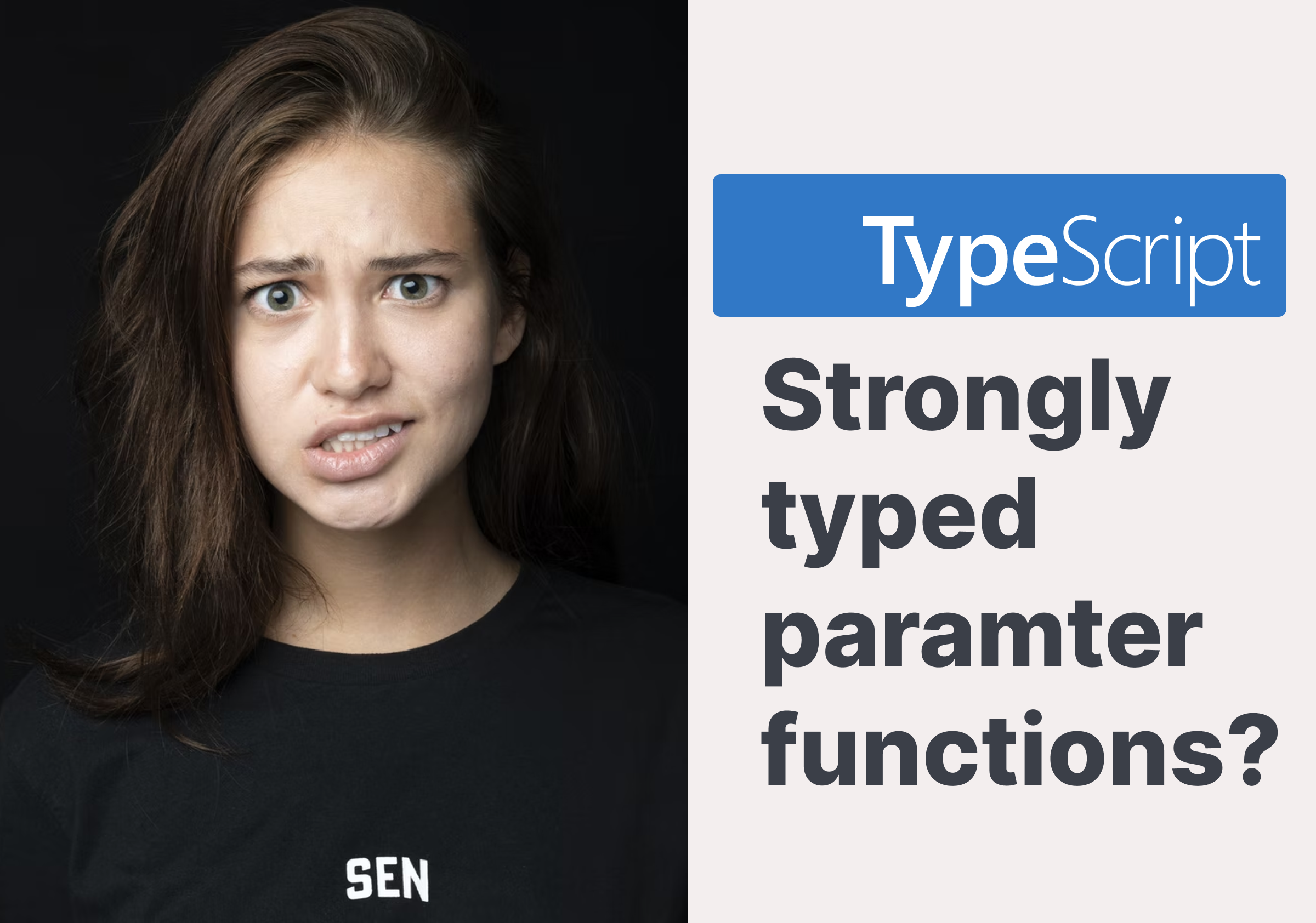
TLDR
This question does not need to be overly explained. The short answer is yes.
Functions can be strongly typed — even as parameters to other functions.
How to use strongly typed function parameters
The accepted answer on this stack overflow post is correct — to a degree.
Assuming you had a function: speak
:
function speak(callback) {
const sentence = "Hello world"
alert(callback(sentence))
}
It receives a callback
that’s internally invoked with a string
.
To type this, go ahead and represent the callback
with a function type alias:
type Callback = (value: string) => void
And type the speak
function as follows:
function speak(callback: Callback) {
const sentence = "Hello world"
alert(callback(sentence))
}
Alternatively, you could also keep the type inline:
function speak(callback: (value: string) => void) {
const sentence = "Hello world"
alert(callback(sentence))
}
And there it is!
You’ve used a strongly typed function as a parameter.
Functions that return any
The accepted answer in the referenced stack overflow post for example says the callback parameter's type must be "function that accepts a number and returns type any"
That’s partly true, but the return type does NOT have to be any
In fact, do NOT use any
.
If your function returns a value, go ahead and type it appropriately:
// Callback returns an object
type Callback = (value: string) => { result: string }
If your callback returns nothing, use void
not any
:
// Callback returns nothing
type Callback = (value: string) => void
Note that the signature of your function type should be:
(arg1: Arg1type, arg2: Arg2type) => ReturnType
Where Arg1type
represents the type of the argument arg1
, Arg2type
the type of the arg2
argument, and ReturnType
the return type of your function.
Conclusion
Functions are the primary means of passing data around in Javascript.
Typescript not only allows you to specify the input and output of functions, but you can also type functions as arguments to other functions.
Go ahead and use them with confidence.
Get a Free Typescript Book?
